Creating a random string on Rails
As we know that in almost all web applications we have to ammend functionalities in which we need to create a random string. This string can contain some alpha numeric characters, numbers, lower case alphabets, upper case alphabets and etc. This random string or token can be generated for many purposes like OTP ( one time password), recovery code etc. Today we will see how to generate an eight character token with the help of arrays. This token will have some numbers, upper case alphabets and lower case alphabets in all jumbled up manner. Here is how we can do it…
token = (('0'..'9').to_a + ('a'..'z').to_a + ('A'..'Z').to_a).shuffle.first(8).join
now as we can see we have taken a variable token in which we are storing numbers from 0 to 9 in the second bracket we have taken all the lower case alphabets. in the third bracket we have taken all the upper case alphabets. and converted them all into array through to_a method. the plus sign concatenates all the arrays into one then finally the .shuffle method will jumble up the whole string and first(8) method will take the first 8 characters from that jumbled up string hence giving us the required token. Now everytime we will call this method it will generate a random string like this as seen in the picture below:
2.2.5 :002 > token = (('0'..'9').to_a + ('a'..'z').to_a + ('A'..'Z').to_a).shuffle.first(8).join
=> "a9bIGCwP"
2.2.5 :003 > token = (('0'..'9').to_a + ('a'..'z').to_a + ('A'..'Z').to_a).shuffle.first(8).join
=> "OZimKuqJ"
2.2.5 :004 > token = (('0'..'9').to_a + ('a'..'z').to_a + ('A'..'Z').to_a).shuffle.first(8).join
=> "c09NCv8Z"
2.2.5 :005 > token = (('0'..'9').to_a + ('a'..'z').to_a + ('A'..'Z').to_a).shuffle.first(8).join
=> "SyVPNqso"
2.2.5 :006 > token = (('0'..'9').to_a + ('a'..'z').to_a + ('A'..'Z').to_a).shuffle.first(8).join
=> "mLhlOeQT"
2.2.5 :007 >
So I hope this blog was helpful to create a random string for multi purposes..!!
Working with GREP in Ruby
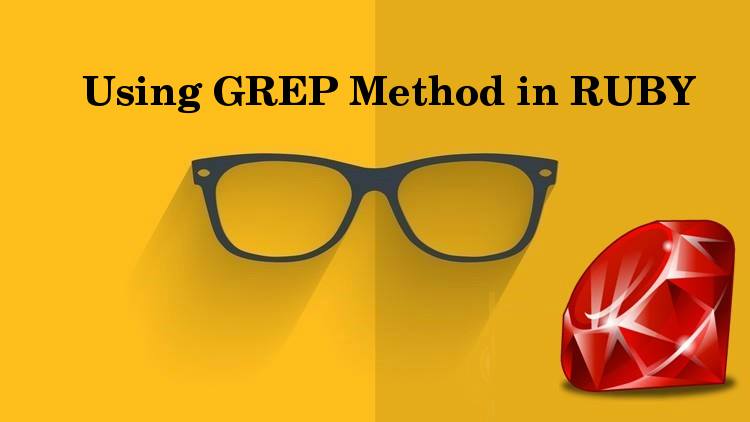
Using grep in RUBY..!!!
As we know that ruby contains a lot of built in modules, in which one of this is the Enumerable module. So before preceddingon what GREP is and How it works, first lets study a little info about Enumerable module.
The Enumerable module provide us a lot of traversal and searching methods which also gives us the ability to do sorting. This module basically provides us simpler methods to manipulate collections with the help of searching, traversing and sorting. The class provides the each method which yields all the successive members of the collection. Enumerable module is included in the class as follows:
class dogs
include Enumerable
def breeds
..
end
..
end
GRIP First of all GREP is a powerful UNIX command. GREP is a fantastic and a very useful method which comes along with the enumerable module in Ruby. Using GREP is very handy and useful when it comes to manipulate collections instead of using a map or select methods. Along with, Grep is very useful in customizing the filtering, lets see how we can customize the filters. Firstly, put the categorization logic as case equal(“===”) in a class once it is done after that the instance of that class will be easily filtered out from an Enumerable object using GREP method. In the ruby methods of enumerable, we use “select” method for filtering and use “map” method for bulk transforming, but grep act as a combination of both these methods.
How does GREP work? To filter the elements in an enumerable object most of the time Select method is usedand it is done by passing a block in it, lets see an example how it is done:
["man", "van", "can", "dude", "pan", "fat"].select{|word| word.include?("an")}
This is a result in
["man","van","can","pan"]
The same thing with less complicated code and syntax can be achieved with this very useful method GREP as follows
["man", "van", "can", "dude", "pan", "fat"].grep(/an/)
which will also return the same result as above but now with a less complicated code. In the above example, what GREP did was it traversed through the array on which it was fired and it took out all the elements which had ‘an’ in them and gave us the resulting array. Grep returns an array for each element in the enum in which the pattern is exactly equal to the elements passed in the array. Grep works with regular expressions and all the other objects such as class and ranges.
Example of GREP are as follows:
[2,20,200,2000].grep(2..200)
# this will result in
# => [2, 20, 200]
In the above example what grep did was it traversed through the array and picked up all the numbers between 2 to 200 and gave us the resulting array.
Lets see one more example
[4,'a',5,'b',6,'c'].grep(Integer)
# This will result in
# => [4,5,6]
the same exampple for string :
[4,'a',5,'b',6,'c'].grep(String)
# This will result in
# => ["a", "b", "c"]
and another example with join
[4,'a',5,'b',6,'c'].grep(String).join
=> "abc"
[4,'a',5,'b',6,'c'].grep(String).join(',')
=> "a,b,c"
In this above example what grep did was it pulled out all the integers from the main array on which we fired the method and gave us all the integers in the resulting array. So, now I hope that the people reading this article would be through with what is GREP, How its used and how useful and handy it is when it comes to manipulate collections.
If you have any inputs or queries please feel free to post in comment section below
RVM Setting the default Ruby
If you would like to make one specific Ruby be the default ruby that is selected when you open a new terminal shell, use the –default flag:
$ rvm --default use 2.1.1
$ ruby -v
ruby 2.1.1p76 (2014-02-24 revision 45161) [x86_64-darwin12.0]
The next time you open a window Ruby 2.1.1 will be the selected ruby.
To switch back to your system ruby:
$ rvm use system
$ ruby -v
ruby 2.0.0p451 (2014-02-24 revision 45167) [universal.x86_64-darwin13]
To switch at any time to the ruby you have selected as default:
$ rvm default
$ ruby -v
ruby 2.1.1p76 (2014-02-24 revision 45161) [x86_64-darwin12.0]
To show what ruby is currently the selected default, if any, do:
$ rvm list
rvm rubies
* ruby-1.9.3-p484 [ x86_64 ]
ruby-2.0.0-p481 [ x86_64 ]
=> ruby-2.1.1 [ x86_64 ]
# => - current
# =* - current && default
# * - default
If you wish to switch back to your system ruby as default, remember that RVM does not “manage” the system ruby and is “hands off”.
This means to set the “system” ruby as default, you reset RVM’s defaults as follows.
$ rvm reset
Note that “default” is merely implemented as an alias with an especially significant name.
If you encounter an error such as “RVM is not a function, selecting rubies with ‘rvm use …’ will not work.”, try using the alias action instead:
$ rvm alias create default 2.1.1
A silly idea to compare Jekyll vs Octopress
Before switching to Jekyll, I tried out Octopress, a blogging framework built on top of Jekyll…. I was really surprised to find out how much I disliked the experience with Octopress. I had an impression that it is a hacker friendly, simple and extensible blog platform, but it was the opposite of that. There are many layers of abstraction, themes are pretty complicated, it comes with many … pre-installed plugins out of the box, dozen depedencies (ruby gems), it uses Sass by default and so on. A fresh copy of Octopress blog consists of staggering 29 directories and 144 files it’s too much..!!
Octopress works almost ok if you are willing to invest plenty of time to customize it or need to build something complex, but it wasn’t my particular use case. The complexity of Octopress defeats the whole purpose of a simple, generated static site. But you know what is “hacker friendly, simple and extensible blog platform”? That’s Jekyll! Jekyll is dead simple and practical. It was a pleasure to setup and customize the default theme. It took less than an hour to have a fully customized blog just the way I wanted. So I am staying with Jekyll this time. The directory structure is really simple and you start with the bare essentials, a simple layout and a CSS file. That’s it!
I tend to prefer simplicity and unix-like philosophy when it comes to choose every day tools for specific tasks. I can always extend, build and combine on top of that, when the time comes.
you can install jekyll with a simple command :
$ gem install jekyll
Check out the Jekyll docs for more info on how to get the most out of Jekyll. File all bugs/feature requests at Jekyll’s GitHub repo. If you have questions, you can ask them on Jekyll Talk.
Viewing the Git log
This list isn’t complete, but the commands shown here are the handful that I use on a day-to-day basis. They should all work in a recent version of git on Linux or macOS (I don’t have access to a Windows computer anymore, but if you know something I don’t, please leave a comment and I’ll amend this article).
git log
: On its own,git log
displays a list of commits and their commit messages in reverse chronological order (most recent commits at the top).git log --reverse
: Display the output in reverse, so the earliest commits appear at the top of the output.git log --oneline
: Passing--oneline
results in a terse, two-column list of commit titles and SHA identifiers.git log -p
: Passing the-p
flag adds a full patch, or diff, to each commit–the code you added and removed.git log -p <filename>
: Passing a file name restricts log output to changes to that file (for example,git log -p app/models/user.rb
). You can remove the-p
flag if you don’t care about the code changes in each commit, or use--oneline
instead to get a quick list. I find that I’m almost always interested in the actual code changes, though. If there’s a chance that git could confuse the filename for a branch name, include--
to disambiguate (git log -p -- app/models/user.rb
).git log -p -S <query>
: Use the-S
flag (also known as the git pickaxe) along with a search term to restrict log output to code changes matching the search (for example,git log -p -S password
). Again, this will work without-p
, or with--oneline
, but this is how I typically use it.git log -p --grep <query>
: The--grep
flag searches only the commit messages for the provided query. Wrap the query in quotes if it contains spaces. (You and your team are hopefully writing useful commit messages!)git log <commit1>..<commit2>
: Restrict output to only the differences between two specific commits by passing them with a..
(and no spaces) between them. This works with SHA identifiers (git log 660bfa2..922b5d2
) as well as branch names (git log rails-4.1..rails-4.2
). Leave either side blank to imply the current branch (git log rails-4.2..
). Use with-p
,-S
, and--grep
as outlined above to filter the results further.git log --stat
: Add a brief list of the files that were altered in each commit, with a count of lines that were added or removed. As you may have guessed by now,--stat
can be used alongside the other flags listed here to narrow results.git log --no-merges
: Omit merge commits from the log output (use--merges
to show only merge commits). I don’t use these as often.
As I mentioned, this is just a list of the tools I use most often to look at the git log. If you want to learn more, Atlassian’s article on advanced git logging is a great next step.
subscribe via RSS